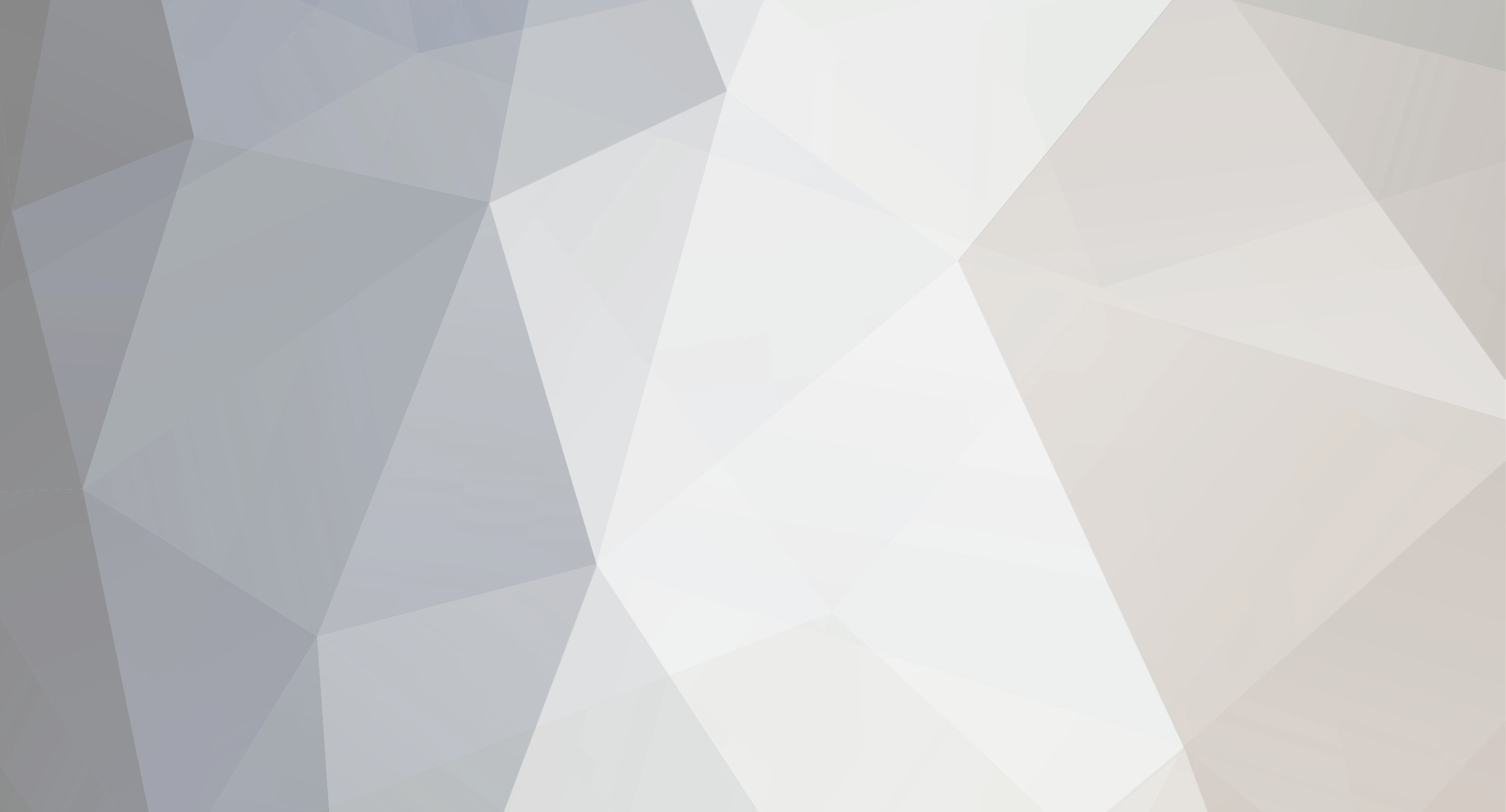
AplexTM
Members-
Content Count
57 -
Last visited
Content Type
Profiles
Forums
Calendar
Gallery
Blogs
Store
Articles
Downloads
Classifieds
Everything posted by AplexTM
-
#!/bin/bash ############################################################################ # # Usage: loc7.sh [options] file ... # # Count the number of lines in a given list of files. # Uses a for loop over all arguments. # # Options: # -h ... help message # -d n ... consider only files modified within the last n days # -w n ... consider only files modified within the last n weeks # # Limitations: # . only one option should be given; a second one overrides # ############################################################################ help=0 verb=0 weeks=0 # defaults days=0 m=1 str="days" getopts "hvd:w:" name while [ "$name" != "?" ] ; do case $name in h) help=1;; v) verb=1;; d) days=$OPTARG m=$OPTARG str="days";; w) weeks=$OPTARG m=$OPTARG str="weeks";; esac getopts "hvd:w:" name done if [ $help -eq 1 ] then no_of_lines=`cat $0 | awk 'BEGIN { n = 0; } \ /^$/ { print n; \ exit; } \ { n++; }'` echo "`head -$no_of_lines $0`" exit fi shift $[ $OPTIND - 1 ] if [ $# -lt 1 ] then echo "Usage: $0 file ..." exit 1 fi if [ $verb -eq 1 ] then echo "$0 counts the lines of code" fi l=0 n=0 s=0 for f in $* do x=`stat -c "%y" $f` # modification date d=`date --date="$x" +%y%m%d` # date of $m days/weeks ago e=`date --date="$m $str ago" +%y%m%d` # now z=`date +%y%m%d` #echo "Stat: $x; Now: $z; File: $d; $m $str ago: $e" # checks whether file is more recent then req if [ $d -ge $e -a $d -le $z ] # ToDo: fix year wrap-arounds then # be verbose if we found a recent file if [ $verb -eq 1 ] then echo "$f: modified (mmdd) $d" fi # do the line count l=`wc -l $f | sed 's/^\([0-9]*\).*$/\1/'` echo "$f: $l" # increase the counters n=$[ $n + 1 ] s=$[ $s + $l ] else # not strictly necessary, because it's the end of the loop continue fi done echo "$n files in total, with $s lines in total"
-
#!/bin/bash # Counting the number of lines in a list of files # for loop over arguments # count only those files I am owner of if [ $# -lt 1 ] then echo "Usage: $0 file ..." exit 1 fi echo "$0 counts the lines of code" l=0 n=0 s=0 for f in $* do if [ -O $f ] # checks whether file owner is running the script then l=`wc -l $f | sed 's/^\([0-9]*\).*$/\1/'` echo "$f: $l" n=$[ $n + 1 ] s=$[ $s + $l ] else continue fi done echo "$n files in total, with $s lines in total"
-
#!/bin/bash # Counting the number of lines in a list of files # function version # function storing list of all files in variable files get_files () { files="`ls *.[ch]`" } # function counting the number of lines in a file count_lines () { f=$1 # 1st argument is filename l=`wc -l $f | sed 's/^\([0-9]*\).*$/\1/'` # number of lines } # the script should be called without arguments if [ $# -ge 1 ] then echo "Usage: $0 " exit 1 fi # split by newline IFS=$'\012' echo "$0 counts the lines of code" # don't forget to initialise! l=0 n=0 s=0 # call a function to get a list of files get_files # iterate over this list for f in $files do # call a function to count the lines count_lines $f echo "$f: $l"loc # store filename in an array file[$n]=$f # store number of lines in an array lines[$n]=$l # increase counter n=$[ $n + 1 ] # increase sum of all lines s=$[ $s + $l ] done echo "$n files in total, with $s lines in total" i=5 echo "The $i-th file was ${file[$i]} with ${lines[$i]} lines"
-
Standalone man-in-the-middle attack framework used for phishing login credentials along with session cookies, allowing for the bypass of 2-factor authentication. evilginx2 is a man-in-the-middle attack framework used for phishing login credentials along with session cookies, which in turn allows to bypass 2-factor authentication protection. This tool is a successor to Evilginx, released in 2017, which used a custom version of nginx HTTP server to provide man-in-the-middle functionality to act as a proxy between a browser and phished website. Present version is fully written in GO as a standalone application, which implements its own HTTP and DNS server, making it extremely easy to set up and use. Installation $GOPATH environment variable is set up properly (def. $HOME/go ). After installation, add this to your ~/.profile , assuming that you installed GO in /usr/local/go : export GOPATH=$HOME/go export PATH=$PATH:/usr/local/go/bin:$GOPATH/bin Then load it with source ~/.profiles . Now you should be ready to install evilginx2. Follow these instructions: sudo apt-get install git make go get -u github.com/kgretzky/evilginx2 cd $GOPATH/src/github.com/kgretzky/evilginx2 make You can now either run evilginx2 from local directory like: sudo ./bin/evilginx -p ./phishlets/ or install it globally: sudo make install sudo evilginx Instructions above can also be used to update evilginx2 to the latest version. [/url]Installing with Docker You can launch evilginx2 from within Docker. First build the container: docker build . -t evilginx2 Then you can run the container: docker run -it -p 53:53/udp -p 80:80 -p 443:443 evilginx2 Phishlets are loaded within the container at /app/phishlets , which can be mounted as a volume for configuration. Installing from precompiled binary packages Grab the package you want fromh ere and drop it on your box. Then do: unzip .zip -d cd If you want to do a system-wide install, use the install script with root privileges: chmod 700 ./install.sh sudo ./install.sh sudo evilginx or just launch evilginx2 from the current directory (you will also need root privileges): chmod 700 ./evilginx sudo ./evilginx USAGE IMPORTANT! Make sure that there is no service listening on ports TCP 443 [color=#000000][size=undefined] , [/size][/color] TCP 80[color=#000000][size=undefined] and [/size][/color] UDP 53[color=#000000][size=undefined] . You may need to shutdown apache or nginx and any service used for resolving DNS that may be running. evilginx2 will tell you on launch if it fails to open a listening socket on any of these ports.[/size][/color] [color=#24292e][size=medium][font=-apple-system, BlinkMacSystemFont, ]By default, evilginx2 will look for phishlets in [/font][/size][/color] ./phishlets/[color=#000000][size=undefined] directory and later in [/size][/color] /usr/share/evilginx/phishlets/[color=#000000][size=undefined] . If you want to specify a custom path to load phishlets from, use the [/size][/color] -p [color=#000000][size=undefined] parameter when launching the tool.[/size][/color] Usage of ./evilginx: -debug Enable debug output -developer Enable developer mode (generates self-signed certificates for all hostnames) -p string Phishlets directory path [color=#24292e][size=medium][font=-apple-system, BlinkMacSystemFont, ]You should see evilginx2 logo with a prompt to enter commands. Type [/font][/size][/color] help[color=#000000][size=undefined] or [/size][/color] help if you want to see available commands or more detailed information on them. Source:https://github.com/kgretzky/evilginx2
-
pftriage is a tool to help analyze files during malware triage. It allows an analyst to quickly view and extract the properties of a file to help during the triage process. The tool also has an analyze function which can detect common malicious indicators used by malware. Changelog 1.0.2 Bug fixes Code clean up Minor updates Added --nobanner option Fix issues with details Update to use file-magic package for libmagic Install Sections Display Section information by using the -s or –sections switch. Additionally, you can pass (-v) for a more verbose view of section details. To export a section pass –dump and the desired section Virtual Address. (ex: –dump 0x00001000) Resources Display resource data by using -r or –resources. To extract a specific resource use -D with the desired offset. If you want to extract all resources pass ALL instead of a specific offset. Imports Display Import data and modules using -i or –imports. Imports which are identified as ordinals will be identified and include the Ordinal used. [*] Exports Display exports using -e or –exports. [*][*] Metadata File and version metadata is displayed if no options are passed on the commandline. [*][*][*] Analyze PFTriage can perform a simple analysis of a file to identify malicious characteristics. [*][*][*][*] Overlay Data Overlay data is identified by analyzing or displaying section information of the file. If overlay data exists PFTriage can either remove the data by using the (–removeoverlay) switch or export the overlay data by using the (–extractoverlay) switch. Source:https://github.com/idiom/pftriage
-
Like to check this out what is :thinking: